Custom control (WPF or Windows Forms) in SOLIDWORKS property Manager Page
Custom control can be assigned to the property in the data model using the CustomControlAttribute and specifying the type of the control to render.
Both Windows Forms controls and WPF controls are supported.
Hosting Windows Forms Control
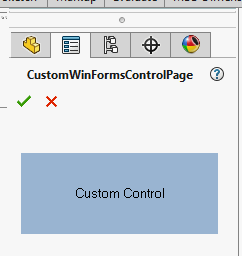
Create a property of any type which will represent data model bound to the control.
public class CustomControlWinFormsModel { public string Text { get; set; } = "Custom Control"; } public class CustomWinFormsControlPage { [CustomControl(typeof(CustomWinFormsControl))] public CustomControlWinFormsModel Model { get; set; } = new CustomControlWinFormsModel(); }
In order to properly associate data model with property manager page, it is required to implement the IXCustomControl interface in the windows forms control.
using System; using System.Windows.Forms; using Xarial.XCad.UI.PropertyPage; namespace Xarial.XCad.Documentation.PropertyPage.Controls { public partial class CustomWinFormsControl : UserControl, IXCustomControl { public event CustomControlValueChangedDelegate ValueChanged; private CustomControlWinFormsModel m_Model; public CustomWinFormsControl() { InitializeComponent(); } public object Value { get { if (m_Model != null) { m_Model.Text = lblMessage.Text; } return m_Model; } set { m_Model = (CustomControlWinFormsModel)value; lblMessage.Text = m_Model.Text; ValueChanged?.Invoke(this, m_Model); } } } }
Framework will bind DataContext property to the corresponding property in the data model of property manager page.
Hosting WPF Control
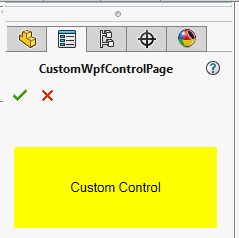
Create a property of any type which will represent data model bound to the control.
public class CustomControlWpfModel : INotifyPropertyChanged { public event PropertyChangedEventHandler PropertyChanged; private string m_Text = "Custom Control"; public string Text { get => m_Text; set { m_Text = value; PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(nameof(Text))); } } } public class CustomWpfControlPage { [CustomControl(typeof(CustomWpfControl))] public CustomControlWinFormsModel Model { get; set; } = new CustomControlWinFormsModel(); }
The value of this property will be automatically assigned to the FrameworkElemet::DataContext property of the control. So it is possible to use WPF binding.
<UserControl x:Class="Xarial.XCad.Documentation.PropertyPage.Controls.CustomWpfControl" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" mc:Ignorable="d" d:DesignHeight="450" d:DesignWidth="800" Background="Yellow"> <Grid> <TextBlock Text="{Binding Path=Text}" VerticalAlignment="Center" HorizontalAlignment="Center" /> </Grid> </UserControl>